스마트시대
1-4.icons and transforms, Reuseable cards 본문
728x90
1. icons and transforms
import 'package:flutter/material.dart';
import 'package:toonflix/widgets/button.dart';
void main() {
runApp(const App());
}
class App extends StatelessWidget {
const App({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
backgroundColor: const Color(0xFF181818),
body: Padding(
padding: const EdgeInsets.symmetric(
horizontal: 20,
),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
const SizedBox(
height: 80,
),
Row(
mainAxisAlignment: MainAxisAlignment.end,
children: [
Column(
crossAxisAlignment: CrossAxisAlignment.end,
children: [
const Text(
'Hey, Selena',
style: TextStyle(
color: Colors.white,
fontSize: 30,
fontWeight: FontWeight.w600),
),
Text(
'Welcome back',
style: TextStyle(
color: Colors.white.withOpacity(0.8),
fontSize: 15,
),
),
],
),
],
),
const SizedBox(
height: 120,
),
Text(
'Total Balance',
style: TextStyle(
fontSize: 22,
color: Colors.white.withOpacity(0.8),
),
),
const SizedBox(
height: 5,
),
const Text(
'\$5 194 482',
style: TextStyle(
fontSize: 48,
fontWeight: FontWeight.w600,
color: Colors.white,
),
),
const SizedBox(
height: 30,
),
// 여기서
Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: const [
Button(
text: 'Transfer',
bgcolor: Color(0xFFF1B33B),
TextColor: Colors.black,
),
Button(
text: 'Request',
bgcolor: Color(0xFF1F2123),
TextColor: Colors.white,
),
],
),
const SizedBox(
height: 100,
),
Row(
crossAxisAlignment: CrossAxisAlignment.end,
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
const Text(
'Wallets',
style: TextStyle(
color: Colors.white,
fontSize: 36,
fontWeight: FontWeight.w600,
),
),
Text(
'View All',
style: TextStyle(
color: Colors.white.withOpacity(0.6),
fontSize: 18,
fontWeight: FontWeight.w600),
),
],
),
const SizedBox(
height: 20,
),
// From here
Container(
clipBehavior: Clip.hardEdge,
decoration: BoxDecoration(
color: const Color(0xFF1F2123),
borderRadius: BorderRadius.circular(25),
),
child: Padding(
padding: const EdgeInsets.all(
30,
),
child: Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
const Text(
'Euro',
style: TextStyle(
color: Colors.white,
fontSize: 30,
fontWeight: FontWeight.w600,
),
),
const SizedBox(height: 10),
Row(
children: [
const Text(
'6 428',
style: TextStyle(
color: Colors.white,
fontSize: 20,
),
),
const SizedBox(
width: 5,
),
Text(
'EUR',
style: TextStyle(
color: Colors.white.withOpacity(0.8),
fontSize: 20),
),
],
),
],
),
Transform.scale(
scale: 2.2,
child: Transform.translate(
offset: const Offset(
8,
15,
),
child: const Icon(
Icons.euro_rounded,
color: Colors.white,
size: 88,
),
),
)
],
),
),
),
],
),
),
),
);
}
}
class MyButton extends StatelessWidget {
const MyButton({
super.key,
});
@override
Widget build(BuildContext context) {
return const mybutton();
}
}
class mybutton extends StatelessWidget {
const mybutton({
super.key,
});
@override
Widget build(BuildContext context) {
return Container(
decoration: BoxDecoration(
color: const Color(0xFF1F2123),
borderRadius: BorderRadius.circular(45),
),
child: const Padding(
padding: EdgeInsets.symmetric(
vertical: 20,
horizontal: 50,
),
child: Text(
'Request',
style: TextStyle(
color: Colors.white,
fontSize: 22,
),
),
),
);
}
}
2. Reuseable cards
import 'package:flutter/material.dart';
import 'package:toonflix/widgets/button.dart';
import 'package:toonflix/widgets/currency_card.dart';
void main() {
runApp(const App());
}
class App extends StatelessWidget {
const App({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
backgroundColor: const Color(0xFF181818),
body: SingleChildScrollView(
child: Padding(
padding: const EdgeInsets.symmetric(
horizontal: 20,
),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
const SizedBox(
height: 80,
),
Row(
mainAxisAlignment: MainAxisAlignment.end,
children: [
Column(
crossAxisAlignment: CrossAxisAlignment.end,
children: [
const Text(
'Hey, Selena',
style: TextStyle(
color: Colors.white,
fontSize: 30,
fontWeight: FontWeight.w600),
),
Text(
'Welcome back',
style: TextStyle(
color: Colors.white.withOpacity(0.8),
fontSize: 15,
),
),
],
),
],
),
const SizedBox(
height: 120,
),
Text(
'Total Balance',
style: TextStyle(
fontSize: 22,
color: Colors.white.withOpacity(0.8),
),
),
const SizedBox(
height: 5,
),
const Text(
'\$5 194 482',
style: TextStyle(
fontSize: 48,
fontWeight: FontWeight.w600,
color: Colors.white,
),
),
const SizedBox(
height: 30,
),
// 여기서
Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: const [
Button(
text: 'Transfer',
bgcolor: Color(0xFFF1B33B),
TextColor: Colors.black,
),
Button(
text: 'Request',
bgcolor: Color(0xFF1F2123),
TextColor: Colors.white,
),
],
),
const SizedBox(
height: 100,
),
Row(
crossAxisAlignment: CrossAxisAlignment.end,
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
const Text(
'Wallets',
style: TextStyle(
color: Colors.white,
fontSize: 36,
fontWeight: FontWeight.w600,
),
),
Text(
'View All',
style: TextStyle(
color: Colors.white.withOpacity(0.6),
fontSize: 18,
fontWeight: FontWeight.w600),
),
],
),
const SizedBox(
height: 20,
),
const CurrencyCard(
name: 'Euro',
code: 'EUR',
amount: '6 428',
icon: Icons.euro_rounded,
isInverted: false,
),
const CurrencyCard(
name: 'Bitcoin',
code: 'BTC',
amount: '9 785',
icon: Icons.currency_bitcoin,
isInverted: true,
),
const CurrencyCard(
name: 'Dollar',
code: 'USE',
amount: '428',
icon: Icons.attach_money_outlined,
isInverted: false,
),
],
),
),
),
),
);
}
}
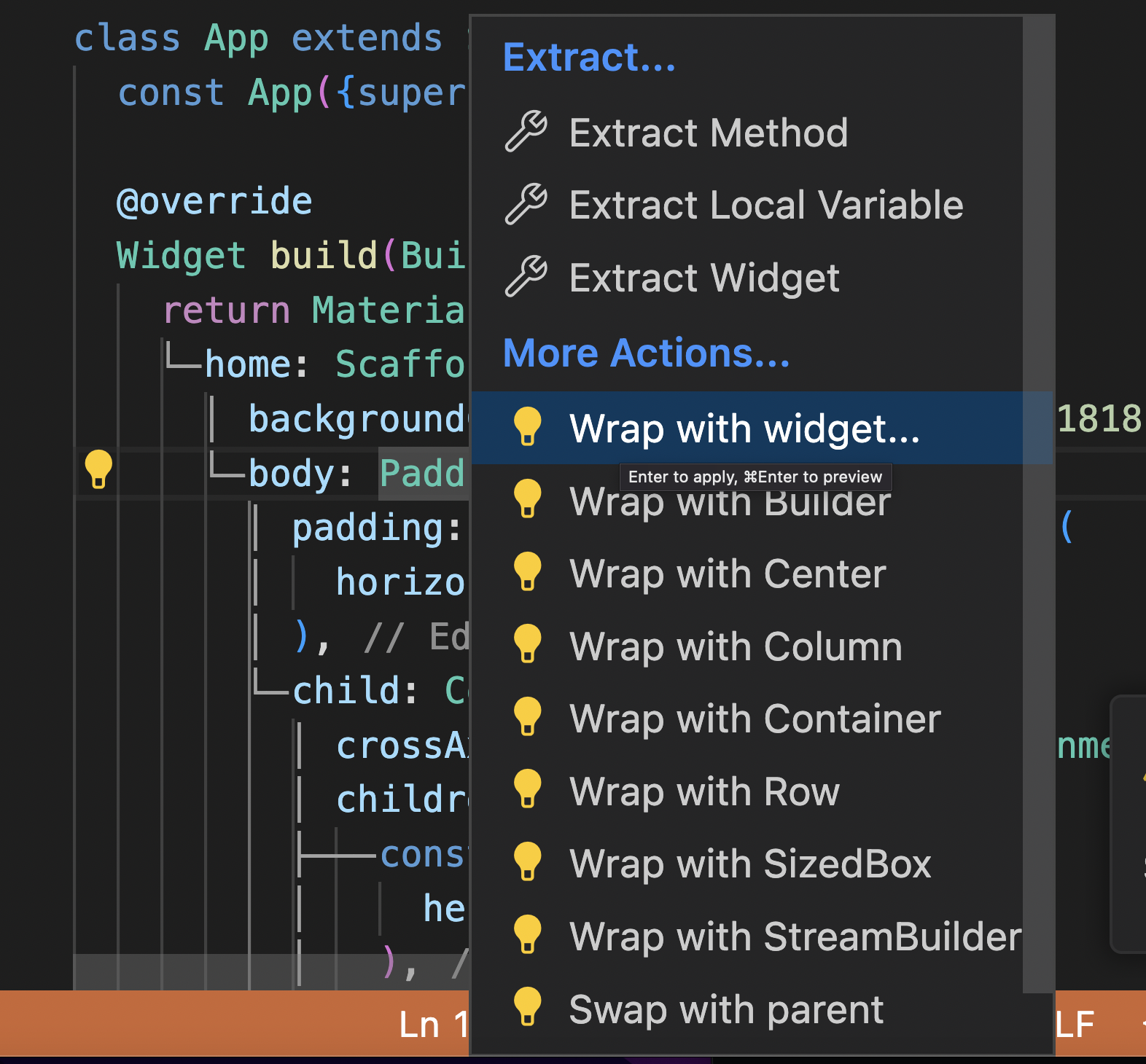
padding을 위젯으로 감싸고
body: SingleChildScrollView(
스크롤 할 수 있게 이 위젯 추가
//currency_card.dart
import 'package:flutter/material.dart';
class CurrencyCard extends StatelessWidget {
final String name, code, amount;
final IconData icon;
final bool isInverted;
final _blackColor = const Color(0xFF1F2123);
const CurrencyCard({
super.key,
required this.name,
required this.code,
required this.amount,
required this.icon,
required this.isInverted,
});
@override
Widget build(BuildContext context) {
return Container(
clipBehavior: Clip.hardEdge,
decoration: BoxDecoration(
color: isInverted ? Colors.white : _blackColor,
borderRadius: BorderRadius.circular(25),
),
child: Padding(
padding: const EdgeInsets.all(
30,
),
child: Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Text(
name,
style: TextStyle(
color: isInverted ? _blackColor : Colors.white,
fontSize: 30,
fontWeight: FontWeight.w600,
),
),
const SizedBox(height: 10),
Row(
children: [
Text(
amount,
style: TextStyle(
color: isInverted ? _blackColor : Colors.white,
fontSize: 20,
),
),
const SizedBox(
width: 5,
),
Text(
code,
style: TextStyle(
color: isInverted
? _blackColor
: Colors.white.withOpacity(0.8),
fontSize: 20),
),
],
),
],
),
Transform.scale(
scale: 2.2,
child: Transform.translate(
offset: const Offset(
8,
15,
),
child: Icon(
icon,
color: isInverted ? _blackColor : Colors.white,
size: 88,
),
),
)
],
),
),
);
}
}
728x90
반응형
'Programing > Flutter' 카테고리의 다른 글
2-1.state (0) | 2023.03.31 |
---|---|
Custom currency widget(currency_card.dart)안에서 offset 관련 parameter 넣기 (0) | 2023.03.28 |
1-3.Reusable Widgets 와 Cards (0) | 2023.03.25 |
1-2.header (0) | 2023.03.18 |
1-1.Hello world build (0) | 2023.03.13 |
Comments